Desktop
3215 Cerberi IX
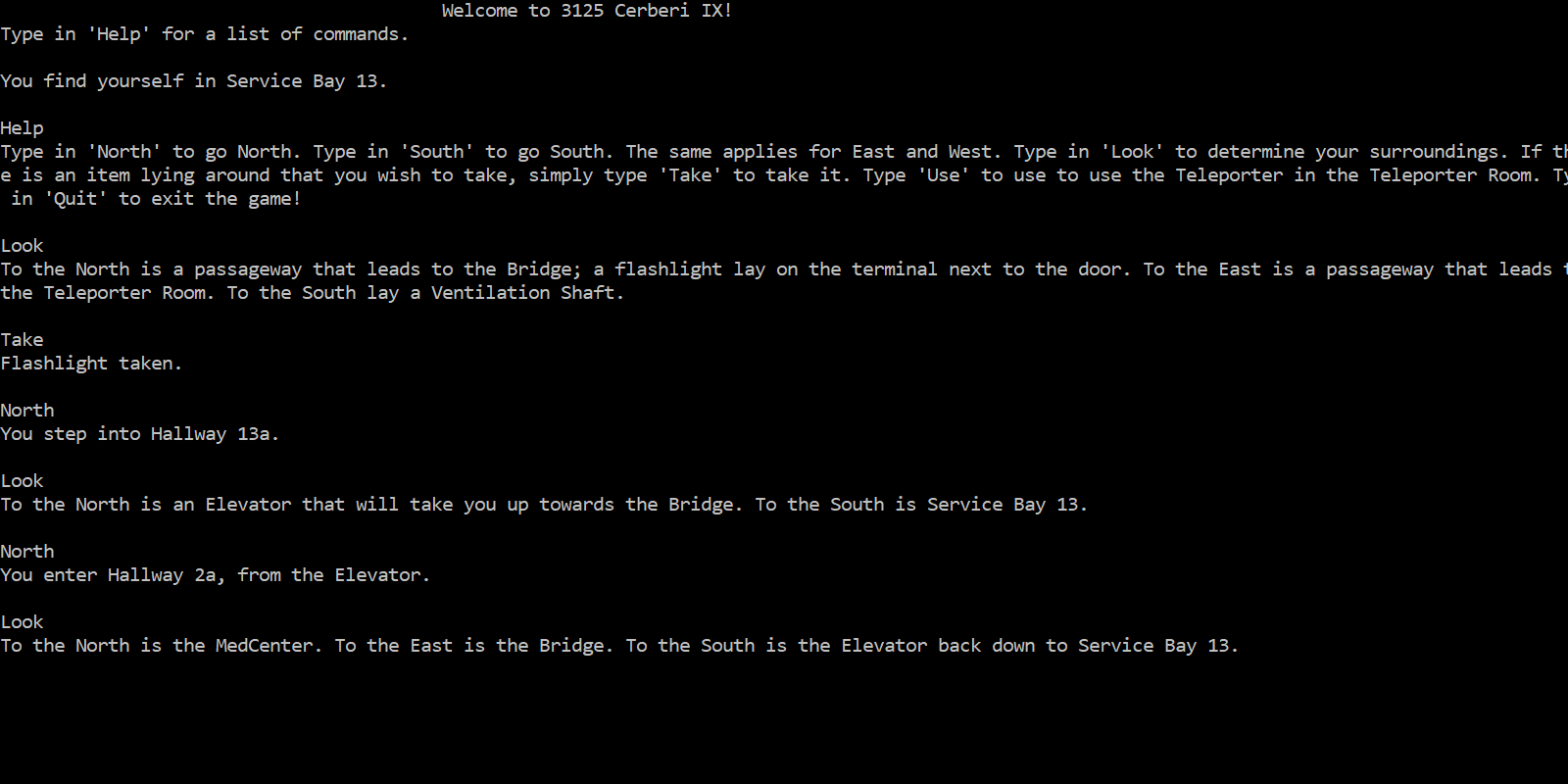
#include "Hero.h" #includeusing namespace std; int main() { //Instantiating a map and player Map GameMap; Hero Player; cout << "\t\t\t\t\t Welcome to 3125 Cerberi IX!\nType in 'Help' for a list of commands.\n\n"; Player.pLocation->GetRoomDesc(); string choice; //Watching player commands while (true) { getline(cin, choice); if (choice == "North" && Player.pLocation->pNorth == 0) { cout << "You cannot go that way.\n\n"; } else if (choice == "North" && Player.pLocation->pNorth != 0) { string rName = Player.pLocation->pNorth->RoomName; if (rName == "Cave Entrance" && Player.hasFlashlight == false) { cout << "The cave is quite dark, and you do not have a flashlight. You do not enter.\n\n"; } else if (rName == "Cave Entrance" && Player.hasFlashlight == true) { Player.pLocation = Player.pLocation->pNorth; Player.pLocation->GetRoomDesc(); } else { Player.pLocation = Player.pLocation->pNorth; Player.pLocation->GetRoomDesc(); if (Player.pLocation->RoomName == "Cave Pit") { break; } else if (Player.pLocation->RoomName == "Dead End") { break; } else if (Player.pLocation->RoomName == "Cliff") { break; } } } else if (choice == "East" && Player.pLocation->pEast == 0) { cout << "You cannot go that way.\n\n"; } else if (choice == "East" && Player.pLocation->pEast != 0) { Player.pLocation = Player.pLocation->pEast; Player.pLocation->GetRoomDesc(); if (Player.pLocation->RoomName == "Cliff") { break; } } else if (choice == "South" && Player.pLocation->pSouth == 0) { cout << "You cannot go that way.\n\n"; } else if (choice == "South" && Player.pLocation->pSouth != 0) { string rName = Player.pLocation->pSouth->RoomName; if (rName == "Landing Zone" && Player.pLocation->RoomName == "Teleporter Room") { cout << "You cannot go that way!\n\n"; } else if (rName == "Ventilation Shaft" && Player.hasScrewDriver == false) { cout << "You need a screwdriver to access this area!\n\n"; } else if (rName == "Ventilation Shaft" && Player.hasScrewDriver == true) { Player.pLocation = Player.pLocation->pSouth; Player.pLocation->GetRoomDesc(); } else { Player.pLocation = Player.pLocation->pSouth; Player.pLocation->GetRoomDesc(); } } else if (choice == "West" && Player.pLocation->pWest == 0) { cout << "You cannot go that way.\n\n"; } else if (choice == "West" && Player.pLocation->pWest != 0) { string rName = Player.pLocation->pWest->RoomName; if (rName == "Ancient Gate" && Player.hasArtifact == false) { cout << "You do not have the artifact needed to open the gate.\n\n"; } else if (rName == "Ancient Gate" && Player.hasArtifact == true) { Player.pLocation = Player.pLocation->pWest; Player.pLocation->GetRoomDesc(); Player.pLocation = Player.pLocation->pNorth; Player.pLocation->GetRoomDesc(); } else { Player.pLocation = Player.pLocation->pWest; Player.pLocation->GetRoomDesc(); } } else if (choice == "Use" && Player.pLocation->RoomName == "Teleporter Room" && Player.hasDossier == false && Player.hasTransponder == false) { cout << "You do not have the required items to leave the ship!\n\n"; } else if (choice == "Use" && Player.pLocation->RoomName == "Teleporter Room" && Player.hasDossier == true && Player.hasTransponder == true) { Player.pLocation = Player.pLocation->pSouth; Player.pLocation->GetRoomDesc(); } else if (choice == "Look") { Player.pLocation->GetRoomLook(); } else if (choice == "Help") { cout << "Type in 'North' to go North. Type in 'South' to go South. The same applies for East and West. Type in 'Look' to determine your surroundings. If there is an item lying around that you wish to take, simply type 'Take' to take it. Type 'Use' to use to use the Teleporter in the Teleporter Room. Type in 'Quit' to exit the game!\n\n"; } else if (choice == "Take" && Player.pLocation->RoomName == "Service Bay 13") { Player.hasFlashlight = true; cout << "Flashlight taken.\n\n"; string nLook = "To the North is a passageway that leads to the Bridge. To the East is a passageway that leads to the Teleporter Room. To the South lay a Ventilation Shaft."; Player.pLocation->m_RoomLook = nLook; } else if (choice == "Take" && Player.pLocation->RoomName == "Engineering Room 13") { Player.hasScrewDriver = true; cout << "Screwdriver taken.\n\n"; string nLook = "To the South is Hallway 13b."; Player.pLocation->m_RoomLook = nLook; } else if (choice == "Take" && Player.pLocation->RoomName == "Research Lab 13") { Player.hasTransponder = true; cout << "Transponder taken.\n\n"; string nLook = "To the West is the Ventilation Shaft you came from."; Player.pLocation->m_RoomLook = nLook; } else if (choice == "Take" && Player.pLocation->RoomName == "MedCenter") { cout << "Stimpaks taken.\n\n"; string nLook = "To the South is Hallway 2a.\n\n"; Player.pLocation->m_RoomLook = nLook; } else if (choice == "Take" && Player.pLocation->RoomName == "Bridge") { Player.hasDossier = true; cout << "Dossier taken. It reads: 'Private: Your assignment for the day is to head to the Teleporter Room, beam down to 3125 Cerberi IX, and retrieve an MIA Sgt that crash landed onto the planet. Take a transponder and immediately let us know when you have made contact with the Sgt. That is all.'\n\n"; string nLook = "To the West is Hallway 2a."; Player.pLocation->m_RoomLook = nLook; } else if (choice == "Take" && Player.pLocation->RoomName == "Cavern") { Player.hasArtifact = true; cout << "Artifact taken.\n\n"; string nLook = "To the West is the Valley.\n\n"; Player.pLocation->m_RoomLook = nLook; } else if (choice == "Quit") { break; } else { cout << "I do not recognize your command. Try adding capitalization to the keyword, and check spelling! Also type 'Help' for a list of available commands!\n\n"; } } cout << "\n\n\t\t\t\t\tA game created by Pierce Smith.\n\n"; system("pause"); return 0; }
Commission Calculator
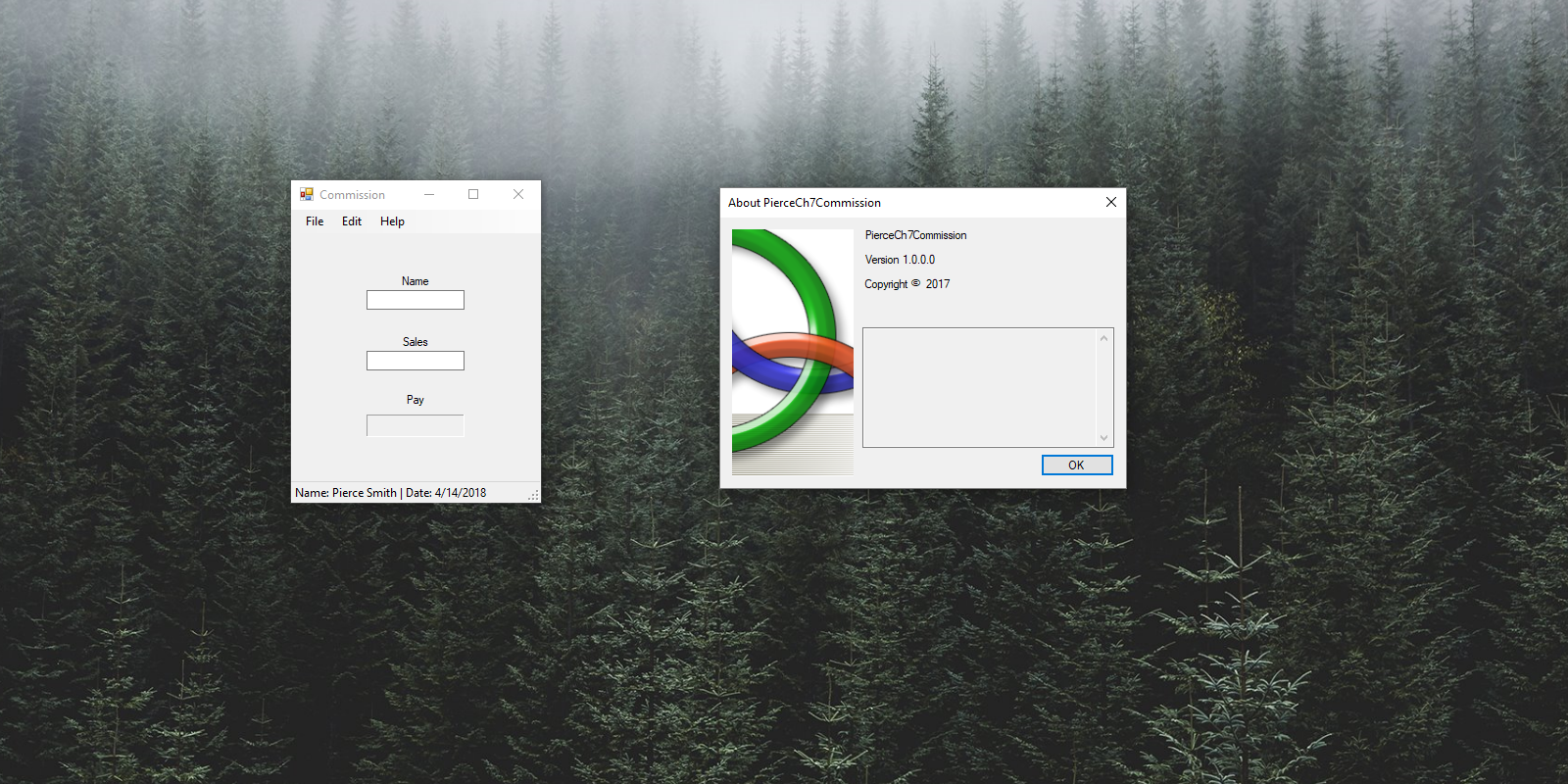
'Programmer: Pierce Smith 'Project: Commission Option Strict On Option Explicit On Public Class Main 'declaration of module variables Public totalSales As Double Public totalPay As Double Public totalCommission As Double Private Sub Form1_Load(sender As Object, e As EventArgs) Handles MyBase.Load 'creating variables for status strip Dim tDay = Today Dim name As String name = "Pierce Smith" slblProgInfo.Text = "Name: " & name & " | Date: " & tDay End Sub Private Sub mnuFilePay_Click(sender As Object, e As EventArgs) Handles mnuFilePay.Click Try If txtName.Text = "" Then MessageBox.Show("Please enter a name in the name field.", "Input Error", MessageBoxButtons.OK, MessageBoxIcon.Error) ElseIf IsNumeric(txtName.Text) Then MessageBox.Show("Please enter alphabetical characters only in the name field.", "Input Error", MessageBoxButtons.OK, MessageBoxIcon.Error) ElseIf txtSales.Text = "" Then MessageBox.Show("Please enter a sales value in the sales field.", "Input Error", MessageBoxButtons.OK, MessageBoxIcon.Error) ElseIf Not IsNumeric(txtSales.Text) Then MessageBox.Show("Please enter a numeric value in the sales field.", "Input Error", MessageBoxButtons.OK, MessageBoxIcon.Error) ElseIf CDbl(txtSales.Text) <= 0 Then MessageBox.Show("Please enter a value that is not negative, and is greater than zero.", "Input Error", MessageBoxButtons.OK, MessageBoxIcon.Error) Else 'good data 'declaration of variables Const basePay As Integer = 250 Dim pay As Double Dim commission As Double 'calling function and assigning to commission variable commission = CalcCom(CDbl(txtSales.Text)) 'calculating the pay pay = basePay + commission 'accumulation totalSales += CDbl(txtSales.Text) totalPay += pay totalCommission += commission 'output lblPay.Text = pay.ToString("C") End If Catch ex As Exception MessageBox.Show("Unexpected Error!", "Error", MessageBoxButtons.OK, MessageBoxIcon.Error) End Try End Sub Private Sub mnuFileSummary_Click(sender As Object, e As EventArgs) Handles mnuFileSummary.Click Summary.ShowDialog() End Sub Private Sub mnuFileExit_Click(sender As Object, e As EventArgs) Handles mnuFileExit.Click Me.Close() End Sub Private Function CalcCom(ByVal sales As Double) As Double 'declaration of variables Const quota As Integer = 1000 Const comRate As Double = 0.15 Dim com As Double 'processing If sales >= quota Then com = sales * comRate Else com = 0 End If 'return value of function Return com End Function Private Sub mnuEditClear_Click(sender As Object, e As EventArgs) Handles mnuEditClear.Click txtName.Text = "" txtSales.Text = "" lblPay.Text = "" txtName.Focus() End Sub Private Sub mnuEditClearAll_Click(sender As Object, e As EventArgs) Handles mnuEditClearAll.Click mnuEditClear_Click(sender, e) totalCommission = 0 totalPay = 0 totalSales = 0 End Sub Private Sub mnuHelpAbout_Click(sender As Object, e As EventArgs) Handles mnuHelpAbout.Click About.ShowDialog() End Sub End Class
Woodlake Pt. I
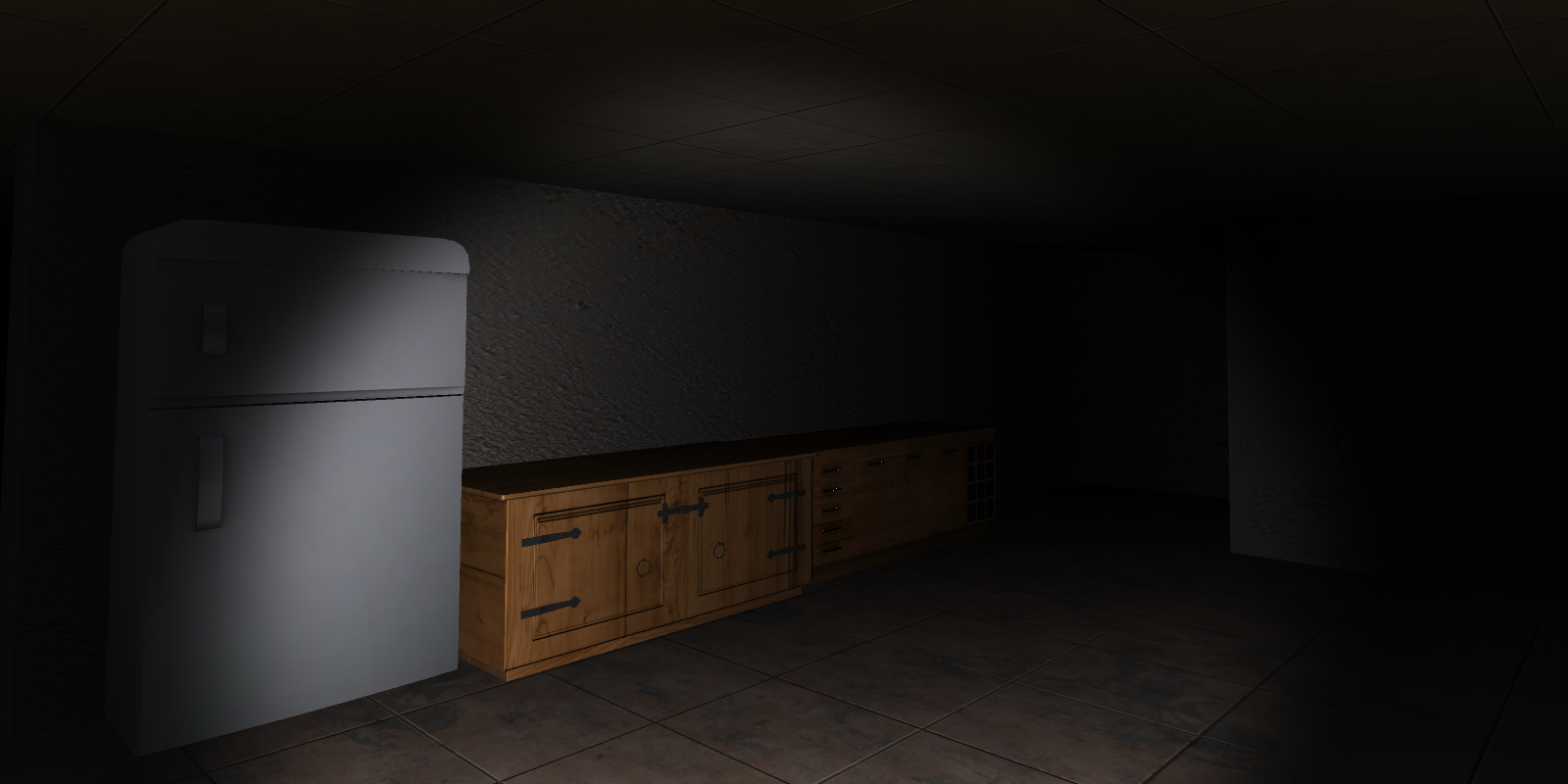
using System.Collections; using System.Collections.Generic; using UnityStandardAssets.Characters.FirstPerson; using UnityEngine.SceneManagement; using UnityEngine; public class KeyPickup : MonoBehaviour { public AudioClip pickUpAudio; public bool pickUpCollide; string pickUpMessage = "Press F to pick up the key."; void OnTriggerEnter(Collider other) { //Telling game to actiavte boolean if(other.gameObject.tag == "Player") { pickUpCollide = true; } } void OnTriggerExit(Collider other) { pickUpCollide = false; } void OnGUI () { if (pickUpCollide == true) { GUI.Label(new Rect((Screen.width / 2) - 40, Screen.height / 2, 400, 200), pickUpMessage); if (Input.GetKeyDown (KeyCode.F)) { GameObject.Find ("FPSController").GetComponent().hasKey = true; GetComponent ().PlayOneShot (pickUpAudio); StartCoroutine (Wait ()); } } } IEnumerator Wait() { yield return new WaitForSeconds (1); Destroy (gameObject); } }